Chart Helper in ASP.NET MVC 3 with Transparent Background
Introduction:
ASP.NET MVC 3 includes some new helper methods that are used for different purposes. Chart helper method is one them, which makes it very easy to create charts in ASP.NET MVC. In this article, I will show you how you can use Chart helper in ASP.NET MVC. I will also show you how to use the themes provided by ASP.NET MVC to style your charts. Then I will show how to create a custom theme for making your chart background as transparent.
Description:
First of all, create a new ASP.NET MVC 3 application. Then add an action method named MyChart to your controller,
01 | public ActionResult MyChart() |
03 | var bytes = new Chart(width: 400, height: 200) |
06 | xValue: new [] { "Math" , "English" , "Computer" , "Urdu" }, |
07 | yValues: new [] { "60" , "70" , "68" , "88" }) |
09 | return File(bytes, "image/png" ); |
Then add an img tag inside your view and set the src attribute to point this action method,
1 | < img src = "@Url.Action(" MyChart")" alt = "SimpleChart" /> |
Now run this application, you will see the following screen,
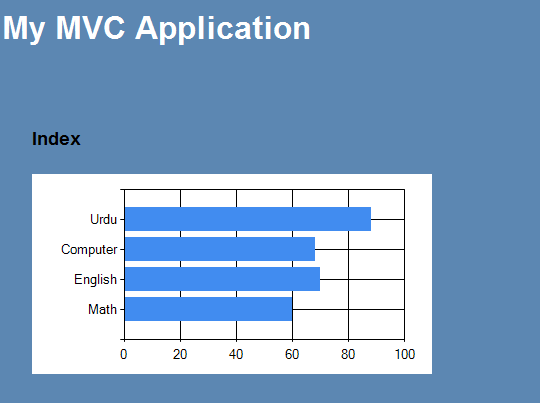
The above screenshot shows you a chart with default theme. Now, add a Yellow theme to your chart,
01 | public ActionResult MyChart() |
03 | var bytes = new Chart(width: 400, height: 200, theme:ChartTheme.Yellow) |
06 | xValue: new [] { "Math" , "English" , "Computer" , "Urdu" }, |
07 | yValues: new [] { "60" , "70" , "68" , "88" }) |
09 | return File(bytes, "image/png" ); |
Run this application again, you will see the following screen,
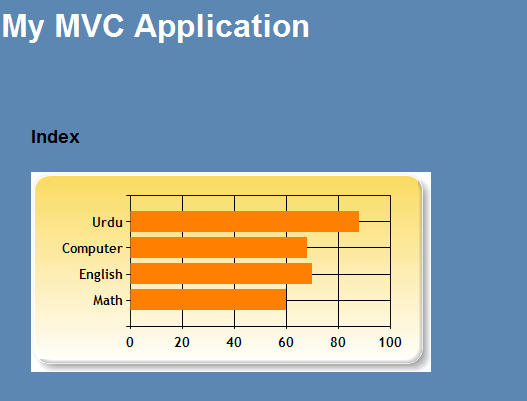
The above screenshot shows you a chart with Yellow theme. This also shows that how easily you can change the theme of your chart. Now, let's add a custom theme which set the background color of your chart to transparent,
01 | public ActionResult MyChart() |
04 | @"<Chart BackColor=""Transparent"" > |
06 | <ChartArea Name=""Default"" BackColor=""Transparent""></ChartArea> |
09 | var bytes = new Chart(width: 400, height: 200, theme: myTheme) |
12 | xValue: new [] { "Math" , "English" , "Computer" , "Urdu" }, |
13 | yValues: new [] { "60" , "70" , "68" , "88" }) |
15 | return File(bytes, "image/png" ); |
Run this application again, you will see the following screen,
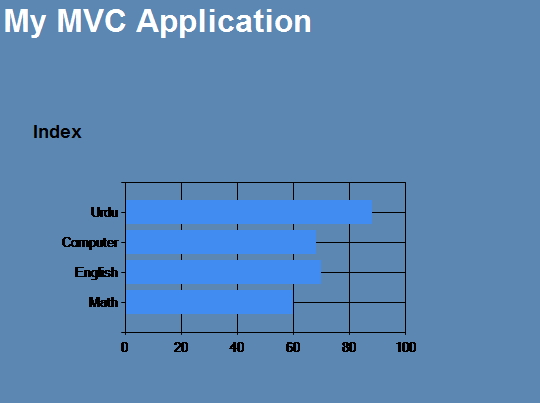
The above screenshot shows you a chart with transparent background color. In addition to changing the background color of your chart, you can also change the gradient, border, rotation, line color, etc. Here are some themes provided by ASP.NET MVC by default, which gives you the idea how to style your charts.
01 | public static class ChartTheme { |
04 | public const string Blue = |
05 | @"<Chart BackColor=""#D3DFF0"" BackGradientStyle=""TopBottom"" BackSecondaryColor=""White"" BorderColor=""26, 59, 105"" BorderlineDashStyle=""Solid"" BorderWidth=""2"" Palette=""BrightPastel""> |
07 | <ChartArea Name=""Default"" _Template_=""All"" BackColor=""64, 165, 191, 228"" BackGradientStyle=""TopBottom"" BackSecondaryColor=""White"" BorderColor=""64, 64, 64, 64"" BorderDashStyle=""Solid"" ShadowColor=""Transparent"" /> |
10 | <Legend _Template_=""All"" BackColor=""Transparent"" Font=""Trebuchet MS, 8.25pt, style=Bold"" IsTextAutoFit=""False"" /> |
12 | <BorderSkin SkinStyle=""Emboss"" /> |
15 | public const string Green = |
16 | @"<Chart BackColor=""#C9DC87"" BackGradientStyle=""TopBottom"" BorderColor=""181, 64, 1"" BorderWidth=""2"" BorderlineDashStyle=""Solid"" Palette=""BrightPastel""> |
18 | <ChartArea Name=""Default"" _Template_=""All"" BackColor=""Transparent"" BackSecondaryColor=""White"" BorderColor=""64, 64, 64, 64"" BorderDashStyle=""Solid"" ShadowColor=""Transparent""> |
19 | <AxisY LineColor=""64, 64, 64, 64""> |
20 | <MajorGrid Interval=""Auto"" LineColor=""64, 64, 64, 64"" /> |
21 | <LabelStyle Font=""Trebuchet MS, 8.25pt, style=Bold"" /> |
23 | <AxisX LineColor=""64, 64, 64, 64""> |
24 | <MajorGrid LineColor=""64, 64, 64, 64"" /> |
25 | <LabelStyle Font=""Trebuchet MS, 8.25pt, style=Bold"" /> |
27 | <Area3DStyle Inclination=""15"" IsClustered=""False"" IsRightAngleAxes=""False"" Perspective=""10"" Rotation=""10"" WallWidth=""0"" /> |
31 | <Legend _Template_=""All"" Alignment=""Center"" BackColor=""Transparent"" Docking=""Bottom"" Font=""Trebuchet MS, 8.25pt, style=Bold"" IsTextAutoFit =""False"" LegendStyle=""Row""> |
34 | <BorderSkin SkinStyle=""Emboss"" /> |
37 | public const string Vanilla = |
38 | @"<Chart Palette=""SemiTransparent"" BorderColor=""#000"" BorderWidth=""2"" BorderlineDashStyle=""Solid""> |
40 | <ChartArea _Template_=""All"" Name=""Default""> |
42 | <MinorGrid Enabled=""False"" /> |
43 | <MajorGrid Enabled=""False"" /> |
46 | <MajorGrid Enabled=""False"" /> |
47 | <MinorGrid Enabled=""False"" /> |
53 | public const string Vanilla3D = |
54 | @"<Chart BackColor=""#555"" BackGradientStyle=""TopBottom"" BorderColor=""181, 64, 1"" BorderWidth=""2"" BorderlineDashStyle=""Solid"" Palette=""SemiTransparent"" AntiAliasing=""All""> |
56 | <ChartArea Name=""Default"" _Template_=""All"" BackColor=""Transparent"" BackSecondaryColor=""White"" BorderColor=""64, 64, 64, 64"" BorderDashStyle=""Solid"" ShadowColor=""Transparent""> |
57 | <Area3DStyle LightStyle=""Simplistic"" Enable3D=""True"" Inclination=""30"" IsClustered=""False"" IsRightAngleAxes=""False"" Perspective=""10"" Rotation=""-30"" WallWidth=""0"" /> |
62 | public const string Yellow = |
63 | @"<Chart BackColor=""#FADA5E"" BackGradientStyle=""TopBottom"" BorderColor=""#B8860B"" BorderWidth=""2"" BorderlineDashStyle=""Solid"" Palette=""EarthTones""> |
65 | <ChartArea Name=""Default"" _Template_=""All"" BackColor=""Transparent"" BackSecondaryColor=""White"" BorderColor=""64, 64, 64, 64"" BorderDashStyle=""Solid"" ShadowColor=""Transparent""> |
67 | <LabelStyle Font=""Trebuchet MS, 8.25pt, style=Bold"" /> |
69 | <AxisX LineColor=""64, 64, 64, 64""> |
70 | <LabelStyle Font=""Trebuchet MS, 8.25pt, style=Bold"" /> |
75 | <Legend _Template_=""All"" BackColor=""Transparent"" Docking=""Bottom"" Font=""Trebuchet MS, 8.25pt, style=Bold"" LegendStyle=""Row""> |
78 | <BorderSkin SkinStyle=""Emboss"" /> |
Note that from these chart themes, Yellow theme used in above example.
Summary:
Chart helper makes it very easy to show chart in your ASP.NET MVC application. In this article, I showed you how to create a chart in ASP.NET MVC. I also showed you how to style a chart and how to change the background color of a chart. Hopefully you will enjoy this article too.