Why don't file uploads work during async postbacks?
As many people have noticed in their AJAX-enabled pages, file uploads do not work when doing an async postback. To my knowledge there's no way to support this scenario due to the browser's security restrictions. To understand why the upload doesn't work it's important to understand how async postbacks are performed and how that's different from how the browser performs a regular postback.
When the browser performs a regular postback it has the benefit of being actual code with no security restrictions running on the client computer. When you submit a form it creates a web request with all the form fields. When it encounters an <input type="file"> it examines the file the user chose, reads it from disk, and adds it to the web request.
When the AJAX JavaScript code is running in the browser it can do the first part about creating a request with all the form fields. However, when it gets to <input type="file"> fields it doesn't have the necessary security permissions to read those files from disk. Imagine what would happen it it could read those files: You could create a page that when visited will upload the user's c:\passwords.txt to a remote server! And we all know that every user has a file called passwords.txt on their C: drive. I bet just now you renamed it so my evil code couldn't run :)
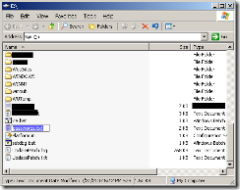
So what's the workaround? Well, quite simply, the workaround is to do anything that doesn't involve a file upload during an async postback. Here are some solutions:
- Have a dedicated "Upload" button that does a regular postback instead of an async postback. You can achieve this using several techniques: Have the button be outside all UpdatePanels; have the button be the target of an UpdatePanel's PostBackTrigger; or call ScriptManager.RegisterPostBackControl() on it.
- Have a dedicated file upload page that doesn't have any UpdatePanels. Many web sites already do this anyway.
And now you might wonder, "why don't ASP.NET AJAX and the UpdatePanel handle this better?" We did give this a fair bit of thought and we decided that doing nothing was the best solution. One option we had was to throw some error from JavaScript if we detected any filled out <input type="file"> tags. But what if you wanted to do an async postback that had nothing to do with the file upload? Or perhaps only detect file uploads in certain regions of the document? That would involve even more expensive DOM tree walks that are already causing some performance degradation with UpdatePanels.
In conclusion, if you want a secure browser, you're not going to get file upload functionality from UpdatePanels. If you don't want a secure browser, please get your head checked. If you want to do file uploads, just use one of the two solutions I provided.
PS: This is the first blog post I've written using Windows Live Writer. I used to use the editor built in to Community Server and was extremely unhappy with it. Live Writer is so easy to use not only for writing and formatting text, but even for adding images to the post. It even creates a nice looking thumbnail automatically with many customization options.