Windows Azure: Major Updates for Mobile Backend Development
This week we released some great updates to Windows Azure that make it significantly easier to develop mobile applications that use the cloud. These new capabilities include:
- Mobile Services: Custom API support
- Mobile Services: Git Source Control support
- Mobile Services: Node.js NPM Module support
- Mobile Services: A .NET API via NuGet
- Mobile Services and Web Sites: Free 20MB SQL Database Option for Mobile Services and Web Sites
- Mobile Notification Hubs: Android Broadcast Push Notification Support
All of these improvements are now available to use immediately (note: some are still in preview). Below are more details about them.
Mobile Services: Custom APIs, Git Source Control, and NuGet
Windows Azure Mobile Services provides the ability to easily stand up a mobile backend that can be used to support your Windows 8, Windows Phone, iOS, Android and HTML5 client applications. Starting with the first preview we supported the ability to easily extend your data backend logic with server side scripting that executes as part of client-side CRUD operations against your cloud back data tables.
With today’s update we are extending this support even further and introducing the ability for you to also create and expose Custom APIs from your Mobile Service backend, and easily publish them to your Mobile clients without having to associate them with a data table. This capability enables a whole set of new scenarios – including the ability to work with data sources other than SQL Databases (for example: Table Services or MongoDB), broker calls to 3rd party APIs, integrate with Windows Azure Queues or Service Bus, work with custom non-JSON payloads (e.g. Windows Periodic Notifications), route client requests to services back on-premises (e.g. with the new Windows Azure BizTalk Services), or simply implement functionality that doesn’t correspond to a database operation. The custom APIs can be written in server-side JavaScript (using Node.js) and can use Node’s NPM packages. We will also be adding support for custom APIs written using .NET in the future as well.
Creating a Custom API
Adding a custom API to an existing Mobile Service is super easy. Using the Windows Azure Management Portal you can now simply click the new “API” tab with your Mobile Service, and then click the “Create a Custom API” button to create a new Custom API within it:
Give the API whatever name you want to expose, and then choose the security permissions you’d like to apply to the HTTP methods you expose within it. You can easily lock down the HTTP verbs to your Custom API to be available to anyone, only those who have a valid application key, only authenticated users, or administrators. Mobile Services will then enforce these permissions without you having to write any code:
When you click the ok button you’ll see the new API show up in the API list. Selecting it will enable you to edit the default script that contains some placeholder functionality:
Today’s release enables Custom APIs to be written using Node.js (we will support writing Custom APIs in .NET as well in a future release), and the Custom API programming model follows the Node.js convention for modules, which is to export functions to handle HTTP requests.
The default script above exposes functionality for an HTTP POST request. To support a GET, simply change the export statement accordingly. Below is an example of some code for reading and returning data from Windows Azure Table Storage using the Azure Node API:
After saving the changes, you can now call this API from any Mobile Service client application (including Windows 8, Windows Phone, iOS, Android or HTML5 with CORS).
Below is the code for how you could invoke the API asynchronously from a Windows Store application using .NET and the new InvokeApiAsync method, and data-bind the results to control within your XAML:
private async void RefreshTodoItems() {
var results = await App.MobileService.InvokeApiAsync<List<TodoItem>>("todos", HttpMethod.Get, parameters: null);
ListItems.ItemsSource = new ObservableCollection<TodoItem>(results);
}
Integrating authentication and authorization with Custom APIs is really easy with Mobile Services. Just like with data requests, custom API requests enjoy the same built-in authentication and authorization support of Mobile Services (including integration with Microsoft ID, Google, Facebook and Twitter authentication providers), and it also enables you to easily integrate your Custom API code with other Mobile Service capabilities like push notifications, logging, SQL, etc.
Check out our new tutorials to learn more about to use new Custom API support, and starting adding them to your app today.
Mobile Services: Git Source Control Support
Today’s Mobile Services update also enables source control integration with Git. The new source control support provides a Git repository as part your Mobile Service, and it includes all of your existing Mobile Service scripts and permissions. You can clone that git repository on your local machine, make changes to any of your scripts, and then easily deploy the mobile service to production using Git. This enables a really great developer workflow that works on any developer machine (Windows, Mac and Linux).
To use the new support, navigate to the dashboard for your mobile service and select the Set up source control link:
If this is your first time enabling Git within Windows Azure, you will be prompted to enter the credentials you want to use to access the repository:
Once you configure this, you can switch to the configure tab of your Mobile Service and you will see a Git URL you can use to use your repository:
You can use this URL to clone the repository locally from your favorite command line:
> git clone https://scottgutodo.scm.azure-mobile.net/ScottGuToDo.git
Below is the directory structure of the repository:
As you can see, the repository contains a service folder with several subfolders. Custom API scripts and associated permissions appear under the api folder as .js and .json files respectively (the .json files persist a JSON representation of the security settings for your endpoints). Similarly, table scripts and table permissions appear as .js and .json files, but since table scripts are separate per CRUD operation, they follow the naming convention of <tablename>.<operationname>.js. Finally, scheduled job scripts appear in the scheduler folder, and the shared folder is provided as a convenient location for you to store code shared by multiple scripts and a few miscellaneous things such as the APNS feedback script.
Lets modify the table script todos.js file so that we have slightly better error handling when an exception occurs when we query our Table service:
todos.js
tableService.queryEntities(query, function(error, todoItems){
if (error) {
console.error("Error querying table: " + error);
response.send(500);
} else {
response.send(200, todoItems);
}
});
Save these changes, and now back in the command line prompt commit the changes and push them to the Mobile Services:
> git add .
> git commit –m "better error handling in todos.js"
> git push
Once deployment of the changes is complete, they will take effect immediately, and you will also see the changes be reflected in the portal:
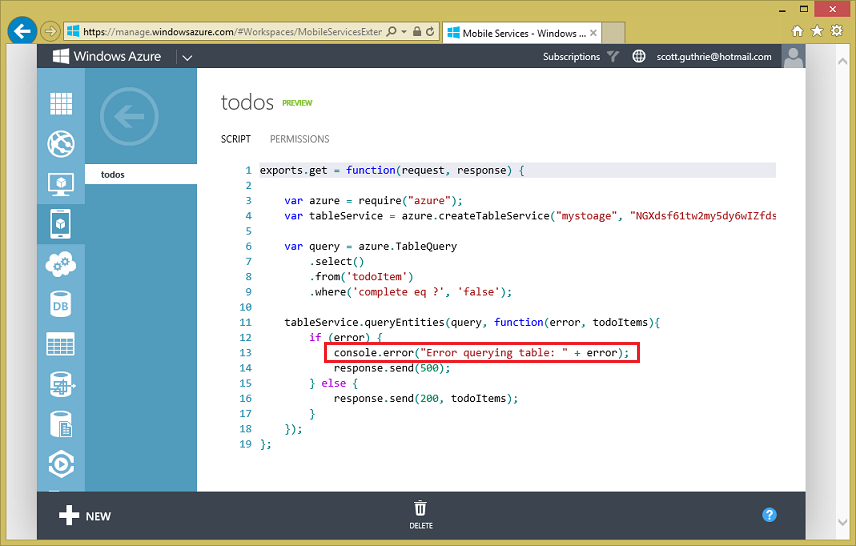
With the new Source Control feature, we’re making it really easy for you to edit your mobile service locally and push changes in an atomic fashion without sacrificing ease of use in the Windows Azure Portal.
Mobile Services: NPM Module Support
The new Mobile Services source control support also allows you to add any Node.js module you need in the scripts beyond the fixed set provided by Mobile Services. For example, you can easily switch to use Mongo instead of Windows Azure table in our example above. Set up Mongo DB by either purchasing a MongoLab subscription (which provides MongoDB as a Service) via the Windows Azure Store or set it up yourself on a Virtual Machine (either Windows or Linux). Then go the service folder of your local git repository and run the following command:
> npm install mongoose
This will add the Mongoose module to your Mobile Service scripts. After that you can use and reference the Mongoose module in your custom API scripts to access your Mongo database:
var mongoose = require('mongoose');
var schema = mongoose.Schema({ text: String, completed: Boolean });
exports.get = function (request, response) {
mongoose.connect('<your Mongo connection string> ');
TodoItemModel = mongoose.model('todoitem', schema);
TodoItemModel.find(function (err, items) {
if (err) {
console.log('error:' + err);
return response.send(500);
}
response.send(200, items);
});
};
Don’t forget to push your changes to your mobile service once you are done
> git add .
> git commit –m "Switched to use Mongo Labs"
> git push
Now our Mobile Service app is using Mongo DB!
Note, with today’s update usage of custom Node.js modules is limited to Custom API scripts only. We will enable it in all scripts (including data and custom CRON tasks) shortly.
New Mobile Services NuGet package, including .NET 4.5 support
A few months ago we announced a new pre-release version of the Mobile Services client SDK based on portable class libraries (PCL).
Today, we are excited to announce that this new library is now a stable .NET client SDK for mobile services and is no longer a pre-release package. Today’s update includes full support for Windows Store, Windows Phone 7.x, and .NET 4.5, which allows developers to use Mobile Services from ASP.NET or WPF applications.
You can install and use this package today via NuGet.
Mobile Services and Web Sites: Free 20MB Database for Mobile Services and Web Sites
Starting today, every customer of Windows Azure gets one Free 20MB database to use for 12 months free (for both dev/test and production) with Web Sites and Mobile Services.
When creating a Mobile Service or a Web Site, simply chose the new “Create a new Free 20MB database” option to take advantage of it:
You can use this free SQL Database together with the 10 free Web Sites and 10 free Mobile Services you get with your Windows Azure subscription, or from any other Windows Azure VM or Cloud Service.
Notification Hubs: Android Broadcast Push Notification Support
Earlier this year, we introduced a new capability in Windows Azure for sending broadcast push notifications at high scale: Notification Hubs.
In the initial preview of Notification Hubs you could use this support with both iOS and Windows devices. Today we’re excited to announce new Notification Hubs support for sending push notifications to Android devices as well.
Push notifications are a vital component of mobile applications. They are critical not only in consumer apps, where they are used to increase app engagement and usage, but also in enterprise apps where up-to-date information increases employee responsiveness to business events. You can use Notification Hubs to send push notifications to devices from any type of app (a Mobile Service, Web Site, Cloud Service or Virtual Machine).
Notification Hubs provide you with the following capabilities:
- Cross-platform Push Notifications Support. Notification Hubs provide a common API to send push notifications to iOS, Android, or Windows Store at once. Your app can send notifications in platform specific formats or in a platform-independent way.
- Efficient Multicast. Notification Hubs are optimized to enable push notification broadcast to thousands or millions of devices with low latency. Your server back-end can fire one message into a Notification Hub, and millions of push notifications can automatically be delivered to your users. Devices and apps can specify a number of per-user tags when registering with a Notification Hub. These tags do not need to be pre-provisioned or disposed, and provide a very easy way to send filtered notifications to an infinite number of users/devices with a single API call.
- Extreme Scale. Notification Hubs enable you to reach millions of devices without you having to re-architect or shard your application. The pub/sub routing mechanism allows you to broadcast notifications in a super-efficient way. This makes it incredibly easy to route and deliver notification messages to millions of users without having to build your own routing infrastructure.
- Usable from any Backend App. Notification Hubs can be easily integrated into any back-end server app, whether it is a Mobile Service, a Web Site, a Cloud Service or an IAAS VM.
It is easy to configure Notification Hubs to send push notifications to Android. Create a new Notification Hub within the Windows Azure Management Portal (New->App Services->Service Bus->Notification Hub):
Then register for Google Cloud Messaging using https://code.google.com/apis/console and obtain your API key, then simply paste that key on the Configure tab of your Notification Hub management page under the Google Cloud Messaging Settings:
Then just add code to the OnCreate method of your Android app’s MainActivity class to register the device with Notification Hubs:
gcm = GoogleCloudMessaging.getInstance(this);
String connectionString = "<your listen access connection string>";
hub = new NotificationHub("<your notification hub name>", connectionString, this);
String regid = gcm.register(SENDER_ID);
hub.register(regid, "myTag");
Now you can broadcast notification from your .NET backend (or Node, Java, or PHP) to any Windows Store, Android, or iOS device registered for “myTag” tag via a single API call (you can literally broadcast messages to millions of clients you have registered with just one API call):
var hubClient = NotificationHubClient.CreateClientFromConnectionString(
“<your connection string with full access>”,
"<your notification hub name>");hubClient.SendGcmNativeNotification("{ 'data' : {'msg' : 'Hello from Windows Azure!' } }", "myTag”);
Notification Hubs provide an extremely scalable, cross-platform, push notification infrastructure that enables you to efficiently route push notification messages to millions of mobile users and devices. It will make enabling your push notification logic significantly simpler and more scalable, and allow you to build even better apps with it.
Learn more about Notification Hubs here on MSDN .
Summary
The above features are now live and available to start using immediately (note: some of the services are still in preview). If you don’t already have a Windows Azure account, you can sign-up for a free trial and start using them today. Visit the Windows Azure Developer Center to learn more about how to build apps with it.
Hope this helps,
Scott
P.S. In addition to blogging, I am also now using Twitter for quick updates and to share links. Follow me at: twitter.com/scottgu